Introduction
The Aerie API uses GraphQL via Hasura to allow for complex querying of any Aerie data. If this is your first time using GraphQL we recommend the following resources:
GraphQL Schema
GraphQL uses a schema to describe the shape of available API data. Aerie employs the Hasura GraphQL engine to generate the Aerie GraphQL API schema. The schema is too large to include in our documentation. If you are running Aerie locally the schema for your Aerie installation can be viewed at http://localhost:8080/console/api/api-explorer (open the "< Docs" tab on the right).
It is important to understand the significance and power of a data-graph based API schema. Below are more detailed resources for helping yourself get familiar with the GraphQL schema and querying capabilities.
Queries & Subscriptions
Mutations
GraphQL Requests
A round trip usage of the API consists of the following three steps:
- Composing a request (query, mutation, or subscription)
- Submitting the request via an HTTP POST request
- Receiving the result as JSON
HTTP POST Request
A standard GraphQL HTTP POST request should use the application/json
content type, and include a JSON-encoded body of the following form:
{
"query": "...",
"operationName": "...",
"variables": { "myVariable": "someValue" }
}
operationName
and variables
are optional fields. The operationName
field is only required if multiple operations are present in the query.
HTTP Response
Regardless of the method by which the query and variables are sent, the response is returned in the body of the request in JSON format. A query’s results may include some data and some errors, and those are returned in a JSON object of the form:
{
"data": {},
"errors": []
}
If there were no errors returned, the "errors"
field is not present in the response. If no data is returned, the "data"
field is only included if the error occurred during execution.
Authentication
To interact with the API you need to be authenticated. For each request Hasura requires an Authorization header to be set, which contains a token in the bearer format: "Bearer YOUR_TOKEN_HERE"
. To get a token you can make an HTTP request to the gateway with your credentials either using the gateway UI, or an HTTP library of your choosing. If you are using Aerie locally you can just use the admin secret and skip using a token.
Gateway UI
To get a token from the gateway UI you can visit http://localhost:9000/#/Auth/post_auth_login, and enter your Aerie username and password (change localhost
do your deployment URL if you are running Aerie on a different domain).
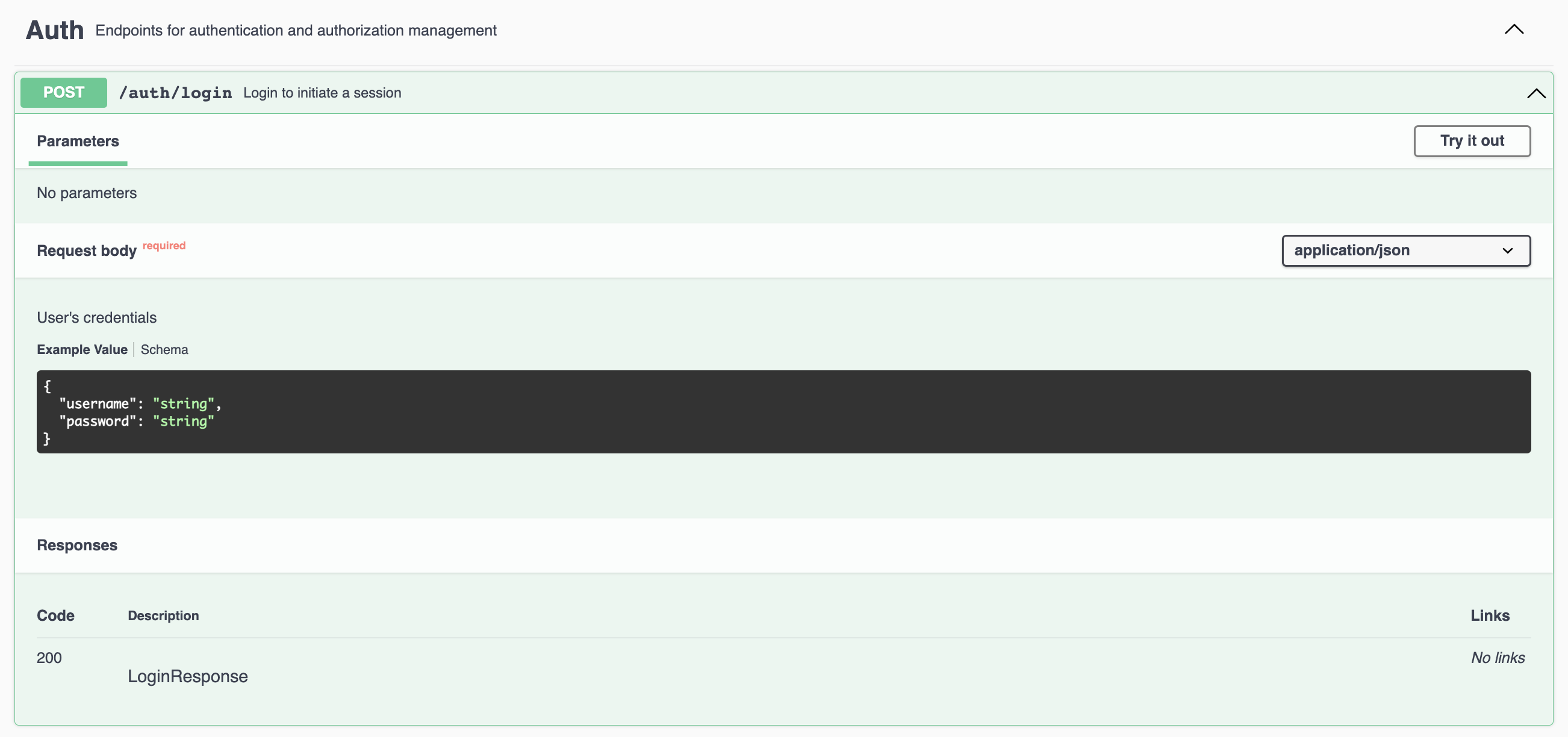
The JSON response contains a token, which you can use in the Authorization
request header in downstream tools to query Hasura.
{
"message": "Login successful",
"success": true,
"token": "YOUR_TOKEN_HERE"
}
Python
You can also query the gateway from Python using the requests library (or any other HTTP library) to obtain a token.
import json
import requests
# Query the gateway to get a token.
response = requests.post(
url='http://localhost:9000/auth/login', # Change from 'localhost' as needed.
json={ 'username': 'YOUR_USERNAME_HERE', 'password': 'YOUR_PASSWORD_HERE' }
)
# Grab the token from the gateway response.
token = response.json().get('token')
# Print the token in Bearer format.
# You can copy and paste this into the 'Authorization' header for Hasura requests.
print(f'Bearer {token}')
Admin Mode
If you are using Aerie locally, or have the admin secret, you can query Hasura without a token and instead set the x-hasura-admin-secret header. This allows you to skip querying the gateway for a token. This is also good if you have a highly-privileged service that needs to query Hasura since it allows access without needing to give the service a user account.
GraphQL Clients
Since a GraphQL API has more underlying structure than a REST API, there are a range of methods by which a client application may choose to interact with the API. A simple usage could use the curl command line tool, whereas a full featured web application may integrate a more powerful client library like Apollo Client or Relay which automatically handle query building, batching and caching.
The Hasura Console
Previously our recommendation was to use the Hasura Console to explore the Aerie API. As of Aerie 1.7.0
the Hasura Console requires the admin secret to access, which makes it unavailable to most for non-local use cases.
If you have the admin secret you can still use the Hasura Console by supplying the secret on the login page.
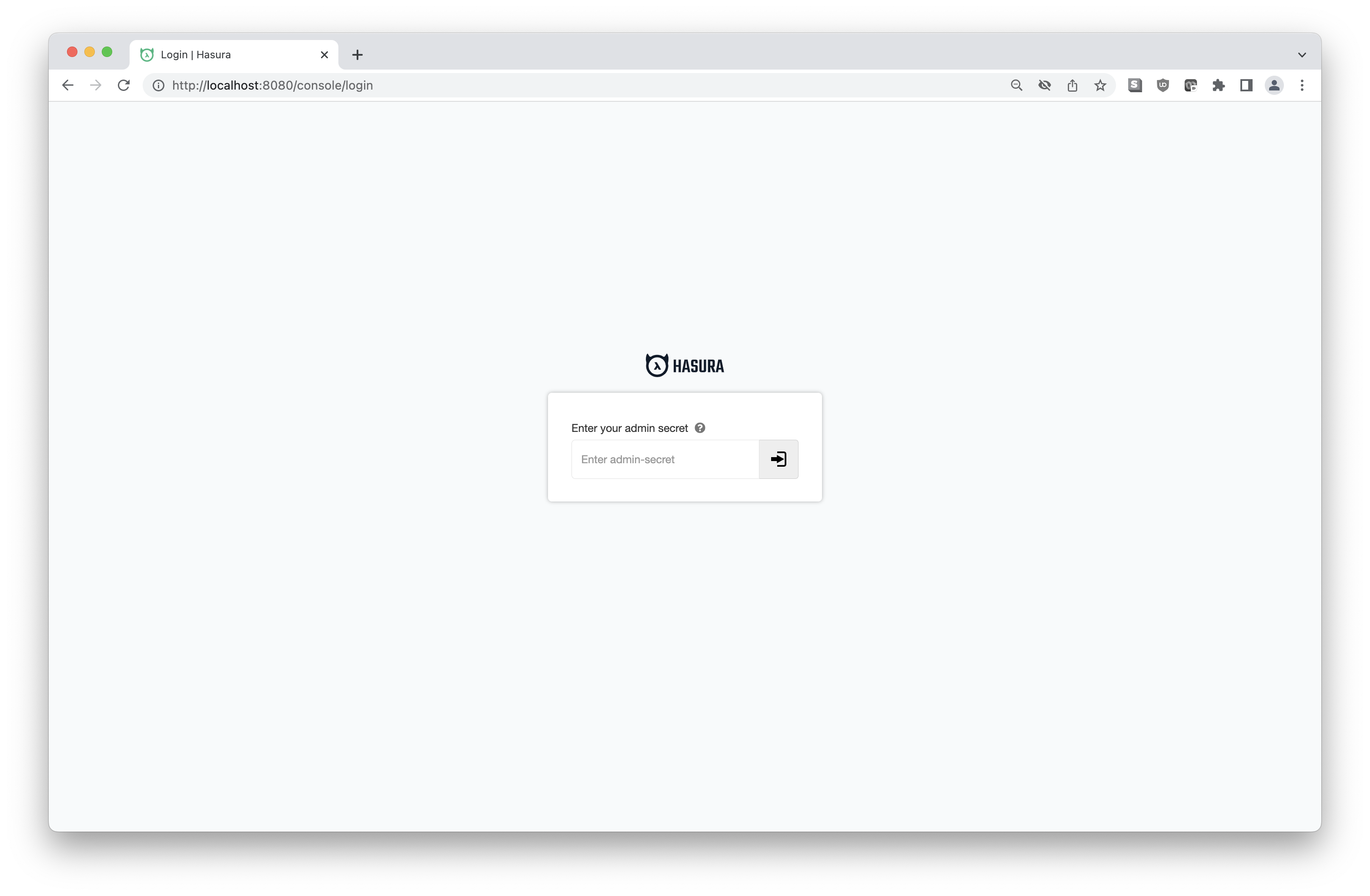
Playground
Aerie provides an API playground via the Altair GraphQL client. You can use the playground user interface to query the Aerie API directly. It is a great way to start exploring Aerie data and get familiar with GraphQL. If you are running Aerie locally you can view the playground (via the gateway server) at http://localhost:9000/api-playground/ (change the localhost
domain as needed).
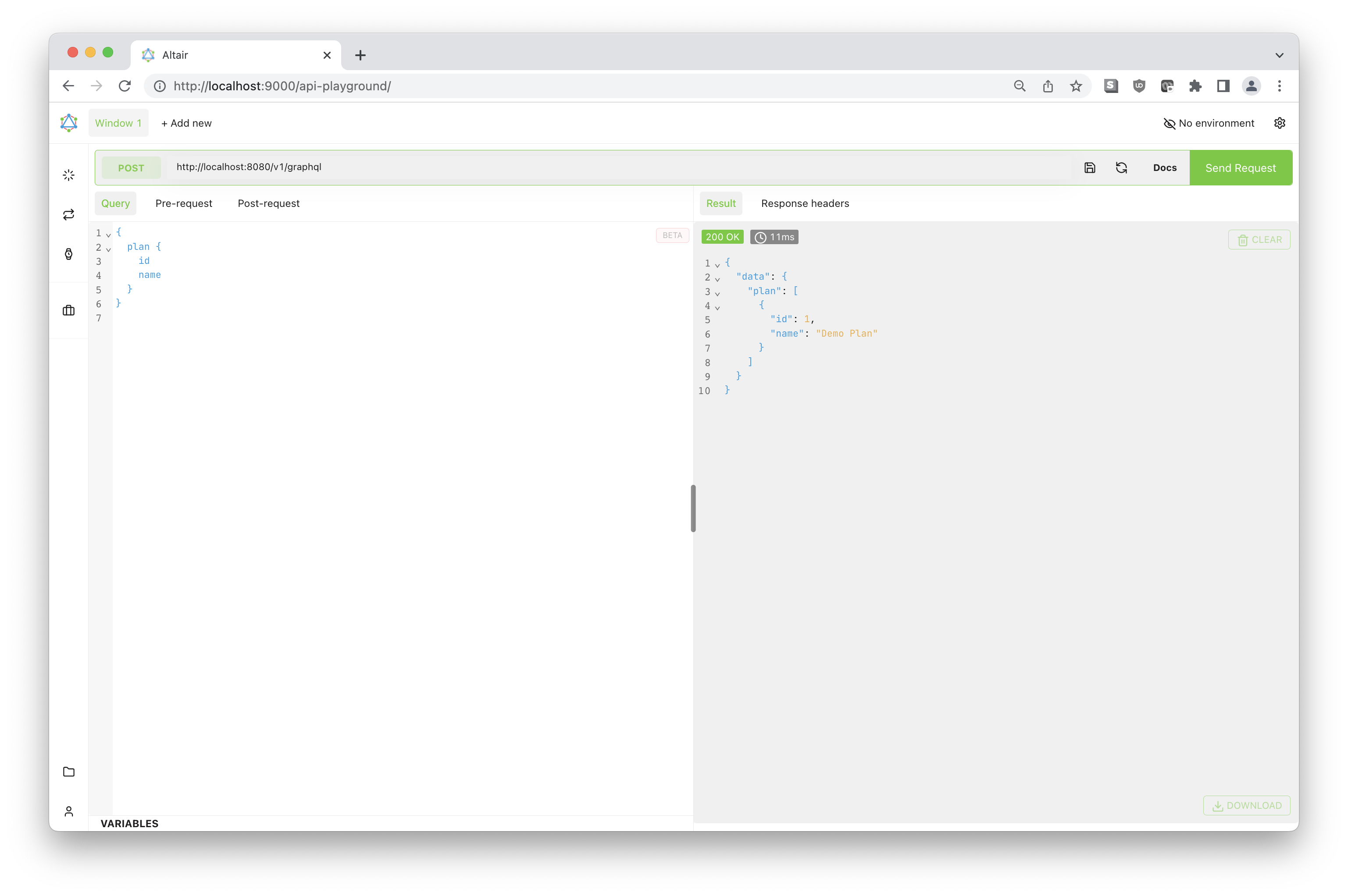
To use the API playground you need to set the proper authorization header (either Authorization
or x-hasura-admin-secret
- see the Authentication section above).
Admin Secret
Access the Global Environment by clicking on "No Environment" -> "Environments..." in the top-right of the page. Set it to the following:
{
"headers": {
"x-hasura-admin-secret": "<YOUR_ADMIN_SECRET>",
"x-hasura-user-id": "<YOUR_AERIE_USERNAME>",
"x-hasura-role": "viewer"
}
}
Authorization
If you do not know the admin secret for your venue, you can instead run a pre-request script to authorize yourself against the Gateway.
For Aerie versions before v2.2.0
- Access the Global Environment by clicking on "No Environment" -> "Environments..." in the top-right of the page. Set it to the following:
{
"headers": {
"Authorization": "Bearer {{user}}",
"x-hasura-role": "viewer"
}
}
In the Query Window, click on Pre-request. Select Enable Pre-request script.
Enter the following pre-request script:
// Fetch a new token from the Gateway
const res = await altair.helpers.request(
'POST',
'/auth/login', // AUTH ENDPOINT OF THE DEPLOYMENT
{
body: { username: '<YOUR_AERIE_USERNAME>', password: '<YOUR_AERIE_PASSWORD>' }, // CREDENTIALS TO LOG IN AS
headers: { 'Content-Type': 'application/json' },
},
);
if (res.success) {
const token = res.token;
await altair.helpers.setEnvironment('user', token);
} else {
altair.log(res);
}
The Playground will now automatically fetch an updated token before each query. To learn more about pre-request scripts, visit the Altair GQL docs.
If you find yourself asked to install the extension altair-graphql-plugin-graphql-explorer
, allow this.
This extension allows you to view and explore the GQL schema by opening the GraphiQL Explorer
using the button midway down the left-side sidebar.
The Explorer is useful for discovering and constructing available queries, mutations, and subscriptions.
In the Query Window, click on "Pre-Request". Find this part of the script:
const res = await altair.helpers.request(
'POST',
'/auth/login', // AUTH ENDPOINT OF THE DEPLOYMENT
{
body: { username: '<YOUR_AERIE_USERNAME>', password: '<YOUR_AERIE_PASSWORD>' }, // CREDENTIALS TO LOG IN AS
headers: { 'Content-Type': 'application/json' },
},
);
Replace <YOUR_AERIE_USERNAME>
and <YOUR_AERIE_PASSWORD>
with the username and password you use to sign in to Aerie.
By default, you will make queries using the viewer
role. If you want to use a different role,
select Set Headers
from the top of the left-side sidebar and update the header x-hasura-role
to a different role you are permitted to use.
To see the list of your permitted roles, use the following query:
{
users_and_roles {
username
hasura_allowed_roles
}
}
After changing your role, you will need to press the Reload Docs
button to the left of the Send Request
button
to update the list of available queries and mutations in the GraphiQL Explorer.
Python
You can query the API in Python using the requests library. Here is a simple example querying for plan data:
import json
import os
import requests
token = os.getenv('AERIE_TOKEN')
response = requests.post(
url='http://localhost:8080/v1/graphql', # Change from 'localhost' as needed.
headers={ 'Authorization': f'Bearer {token}' }, # See 'Authorization' section above to get this.
json={ 'query': 'query { plan { id } }' }
)
print(json.dumps(response.json(), indent=2))
If you have the admin secret you can alternatively use it to make your request. Set the x-hasura-admin-secret
header (default admin secret shown below):
headers={ 'x-hasura-admin-secret': 'aerie' }
Command Line
One may build and send a query or mutation via any means that enable an HTTP POST request to be made against the API. For example, this can be easily done using the command line tool graphqurl. There is also a community maintained tool called aerie-cli that exposes common Aerie queries (e.g. create plan, run simulation, etc.) on the command line. It is a good option if you need to automate common tasks without implementing queries yourself.
Browser Developer Console
Requests can also be tested using JavaScript from a web-browser (or Node.js). For example the following JavaScript can be used to make a simple query using the fetch API:
const response = await fetch('http://localhost:8080/v1/graphql', {
body: JSON.stringify({ query: 'query { plan { id } }' }),
headers: {
Authorization: `Bearer YOUR_TOKEN_HERE`, // See the 'Authentication' section above to get this.
'Content-Type': 'application/json',
},
method: 'POST',
});
const data = await response.json();
console.log(data);
Here is an example of a possible shape of the data logged to the console:
{
"data": {
"plans": [{ "id": 1 }, { "id": 2 }]
}
}
This JavaScript can then be used as a hard-coded query within a client tool/script. For more complex and dynamic interactions with the Aerie API it is recommended to use a GraphQL client library.
Client Libraries
When developing a full featured application that requires integration with the Aerie API it is advisable that the tool make use of one of the many powerful GraphQL client libraries like Apollo Client or Relay. These libraries provide an application functionality to manage both local and remote data, automatically handle batching, and caching.
In general, it will take more time to set up a GraphQL client. However, when building an Aerie integrated application, a client library offers significant time savings as the features of the application grow. One might choose to begin using HTTP requests as the client's API integration mechanism and later switch to a client library as the application becomes more complex.
GraphQL clients exist for the following programming languages:
- C# / .NET
- Clojurescript
- Elm
- Flutter
- Go
- Java / Android
- JavaScript
- Julia
- Kotlin
- Swift / Objective-C iOS
- Python
- R
A full description of these clients is found at https://graphql.org/code/#language-support